Python is one of the most user friendly and easy to learn programming languages suited both for programmers as well as developers. If you are planning for a career in Machine Learning, Artificial Intelligence and Data Science or Analytics then Python is the best suited for you.
Google’s search algorithm is powered by Python, Youtube Video Processing and Data Analytics is handled using Python. Companies like Facebook and Spotify use it to handle the user generated content and data.
Also, unlike other programming languages Python is known for its clean and easy syntax. It does more in less code. If you know a bit of programming then you probably remember that most programming languages use semicolons to end a line or block of code. However, You don’t need to use semicolons (;) at the end of every code block if you are programming in Python.
Also Python makes the code structure visually clear as it makes it mandatory to follow indentation which would come as a surprise, if you have never programmed in languages like Python, Haskell or F#. We would be explaining indentation errors and other such common mistakes which most beginners make when programming in Python.
Install Python
Before writing your first python program you would have to install it on your computer. For steps to install Python on your Windows computer you can visit the following link.
Write ‘Hello World’ in Python.
After installing Python. Open the Notepad app in Windows or any code editor like Visual Studio Code or Notepad++.
We would be using the Notepad app which is available on all Windows computers. Open the Notepad application and write the following line of code.
print(“Hello World!”)
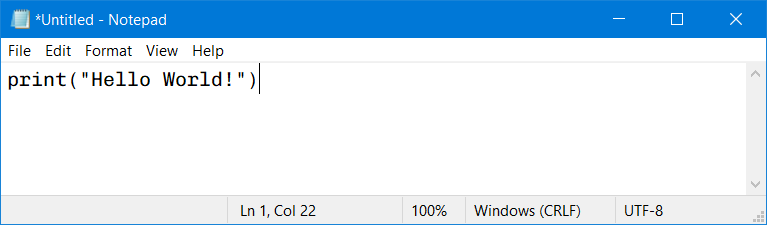
Then press Ctrl + S to open the Save dialog. In the Save as type drop down select All Files (*.*).
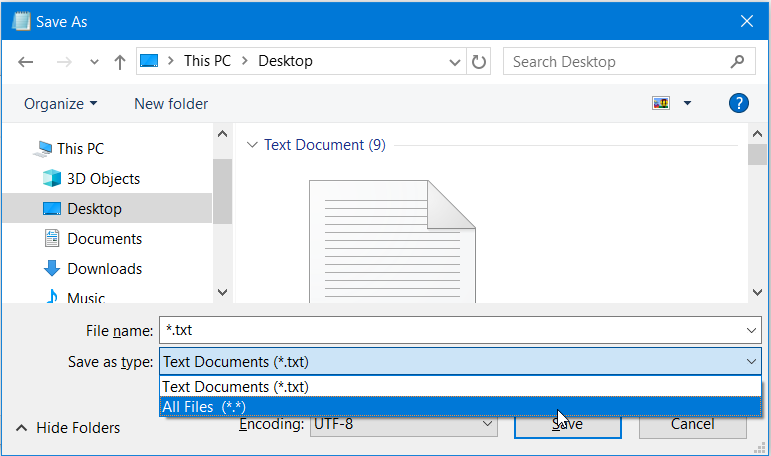
Name the file hello.py (.py is the extension for python files). Choose the Desktop for the save location.
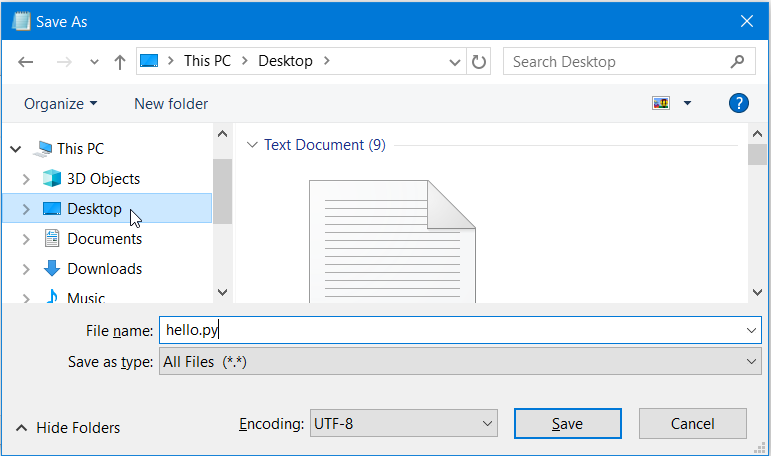
You have successfully created your first program in python which would print the “Hello World!” message when executed.
How to execute the Python Program?
Python programs can be executed from the command line in Windows. You can open the Command Prompt app to execute the hello.py program which we just created.
In the Command Prompt window we would have to type python followed by the path of the python program.
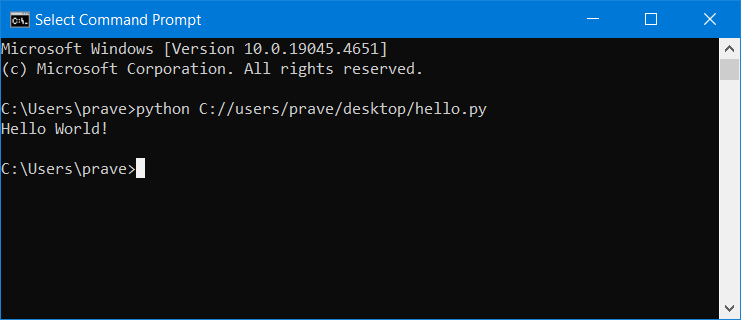
The other way of doing this is to change the directory to Desktop (or the directory where you have saved your hello.py file) in the Command Prompt.
To change the directory to desktop, simply type the following command, after replacing the <your_username> with your actual windows username, in the Command Prompt window.
cd C://Users/<your_username>/desktop
Then type python followed by hello.py. This would execute the program in the same way and removes the hassle of typing the full path of the hello.py python program.
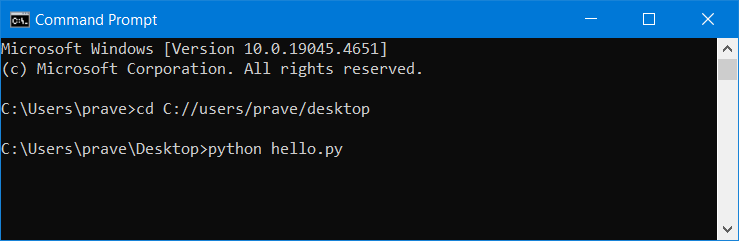
After writing your first python program you may have noticed that it doesn’t use a semicolon at the end of every line of code.
Don’t Forget Indentation in Python
Python is a bit of a neat freak when it comes to formatting. Other programming languages do not bother with indentation and formatting of the code. You can write the code without giving a damn about indentation and it would still work.
Python, however, would throw an indentation error when you don’t follow this guideline or rule. Let’s explain this with the help of an example. Let’s modify the hello.py code which we just created.
Delete everything in hello.py and place the following lines of code and save hello.py
print("Enter a number:")
num = int(input())
if num%2 == 0:
print("It's an even number!")
else:
print("It's an odd number!")
The code asks the user for a number and checks whether it’s an odd or even number and accordingly displays a message.
Execute this program by typing the following lines of code in the Command Prompt window. Make sure to replace <username> with your windows username.
cd C://users/<username>/desktop
python hello.py
You would notice that Python throws an indentation error.
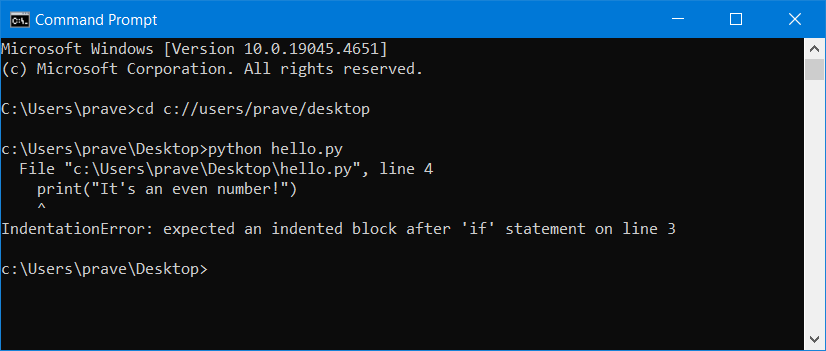
Now open hello.py in Notepad, and give a single space before each print( ) statement as shown below, and save hello.py
print("Enter a number:")
num = int(input())
if num%2 == 0:
print("It's an even number!")
else:
print("It's an odd number!")
Now, execute the program again by typing the following in the command prompt window. This time the code would execute as expected.
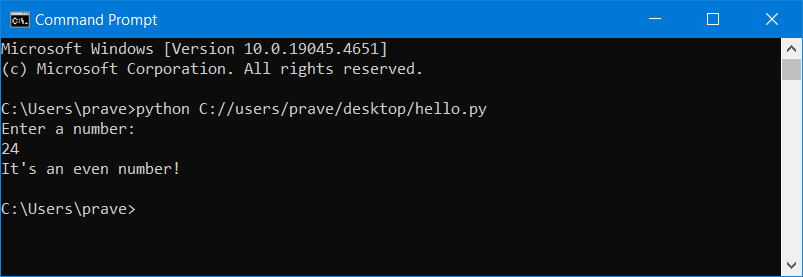
So, in python you would have to make sure that you are following indentation and also make sure that it is consistent. If you give a single space in the first print( ) statement then put the same number of spaces in the second print( ) statement as well. You can also press tab before writing the print( ) statement, which would make sure that you are following the indentation rule.
You can also use a code editor program like Notepad ++ or Visual Studio Code that would automatically add the spaces or indentation for you.
So, now you have installed Python and run your first program.