QR codes make it a lot easier to share URLs, social media links, make payments and share contact information like phone numbers and vCards. You scan them with a camera enabled device, like your smartphone and tablet and they would display the information contained within.
However, making them is not so easy and only a specialized tool or app can make them. If you are a Python programmer then this method would teach you to create a QR code with any alphanumeric information.
Even if you don’t have any experience in Python or programming; you can still follow the instructions given here to create QR codes. You can create simple hyperlinks or social media links using this method.
What would you need?
A Computer with Python installed and a working internet connection. If you don’t have Python installed then you can install it using the method explained in the following link.
https://digitional.com/how-to-install-the-latest-version-of-python-in-windows
How to make the QR Code in Python?
Install Python
You can install Python by following the instructions in the link given below.
https://digitional.com/how-to-install-the-latest-version-of-python-in-windows
After installing Python we would have to install a module or package named PyQRCode. This module can be downloaded from the command line using the pip command.
Install the Modules for making the QR Code
If you are running Python on a Windows computer then open the Command Prompt window and type the following command to install the module. (Also, make sure that you are connected to the internet before executing this command.
pip install pyqrcode
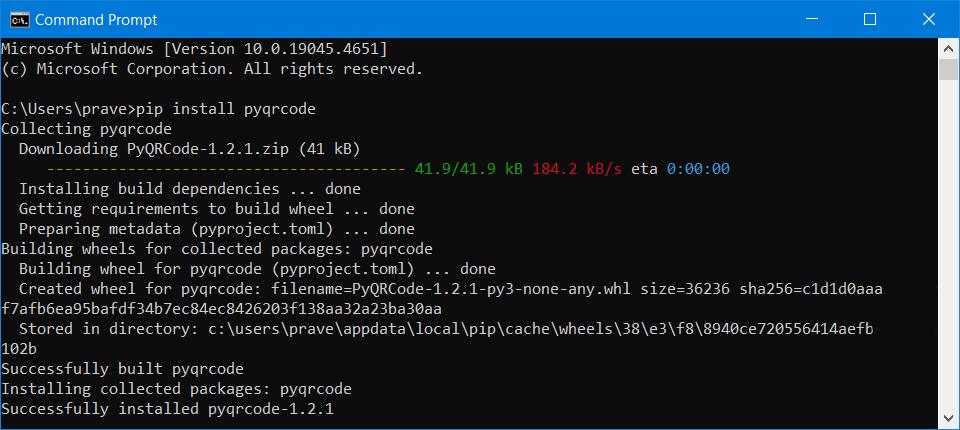
After that we would need a module which would convert the output of the PyQRCode module into a PNG image. The module is called pypng and you can install it by typing the following command in the Command Prompt window.
pip install pypng
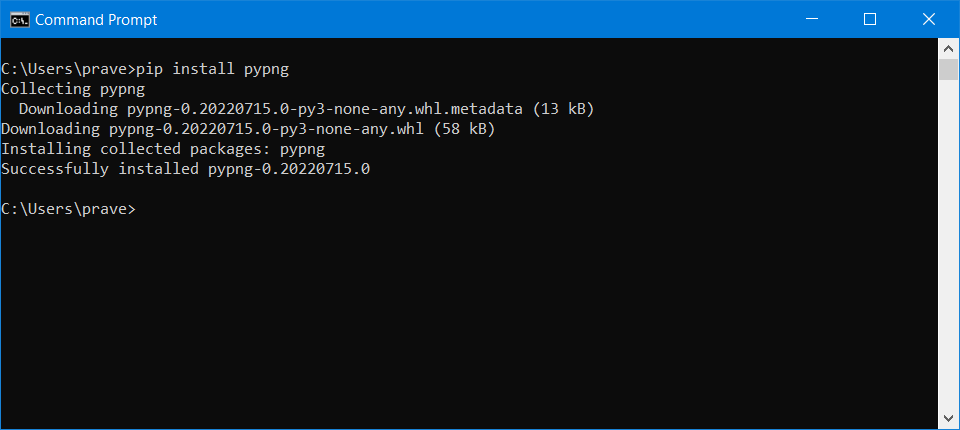
Write The Python Program for Making the QR Code
After installing these modules we would write the Python program to create the QR Code which would contain the information provided by us. If you have never written or run a Python program before then read the following article which would teach you to write your first python program and execute it.
Now, we will write the Python program to make the QR code. You can write Python code in any code editor program like Visual Studio Code or Notepad++. However, we have used Notepad which is available on every Windows Computer.
Open a Notepad window. The first thing which we would write is the code to import the PyQRCode module in our qrcode.py program, using the Python’s import
directive. Then we would also import the pypng Python module so that we can write the output as a PNG image file.
import pyqrcode
import png
Then we would assign the URL or link that we want to encode in the QR code to the variable qrstring. The QR code would contain this information and display the link when scanned using a smartphone or camera enabled device. We have put the URL of our website in the qrstring variable.
qrstring = "www.digitional.com"
Then we would pass this string to the pyqrcode module which would generate the QR data which is then assigned to the url variable.
url = pyqrcode.create(qrstring)
The next line of code would then convert the data to a PNG image format and write it to qrcode.png file.
url.png('qrcode.png', scale = 20)
The scale parameter determines the dimensions of the PNG image file. You can set it to 10 if you want a small image and make it 20 if you want a high resolution image. You can set it to any number between 1 and 40.
Your, final code should look like this,
import pyqrcode
import png
qrstring = "www.digitional.com"
url = pyqrcode.create(qrstring)
url.png('qrcode.png', scale = 20)
Then, save this Python code as a qrocde.py file after selecting All Files (*.*) in the Save as type dropdown in the Notepad’s Save As dialog. Save the program on your desktop.
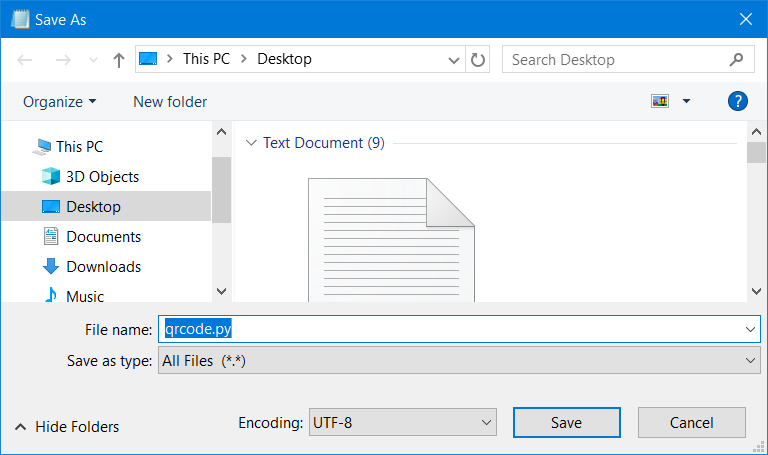
Execute the Python Program
Now, all you have to do is execute this python program which we just created and the PNG image containing the QR would be generated in the folder where your Python program is located. In our case it’s the desktop.
To execute the program open the Command Prompt window and type the following line of code after replacing the <username> with your own Windows username.
cd C:/users/<username>/desktop/
This will make your desktop as the working directory. Then you can execute the the qrcode.py python file by writing the following command.
python qrcode.py
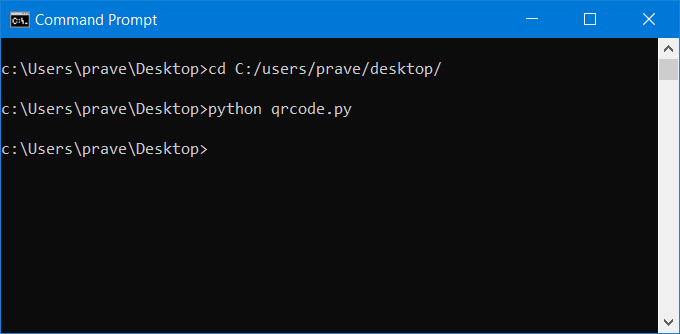
This will generate the QR Code and create a qrcode.png file on your desktop. Open the PNG image and scan it with any camera enabled device or smartphone and it would display a clickable link with the text we provided in the python program.
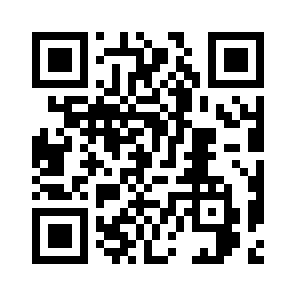
You can modify the program to create another link. Moreover, PyQRCode can also generate the image in SVG and EPS file formats. These file formats are size independent and can be scaled to any size without losing resolution or quality. Here’s the modified program to create the QR code in the SVG file format.
import pyqrcode
import png
qrstring = "www.digitional.com"
url = pyqrcode.create(qrstring)
url.svg("qrcode.svg", scale = 8)
If you are looking for advanced options then you can also pass the following parameters. The parameters passed in the following code would pass error correction, version and mode parameters for the QR code.
url = pyqrcode.create(qrstring, error='L', version=27, mode='binary')
What is the error correction parameter?
QR codes can be scanned even when dirty or deformed. This is achieved by using error correction algorithms which generate additional information from the data that is then encoded along with the QR code data. This additional information would make the QR readable even when there are missing dots. However, this increases the number of dots and file size of your QR image.
What is the version of the QR code?
QR code versions range from 1 to 40, and each version has a different number of modules, or black and white dots, that make up the code. Version 1 has 21 × 21 modules and Version 40 has 177 × 177 modules. Again, increase this number and your QR would become more complex and contain more information.
What is the mode of the QR code?
The mode of the QR code specifies the kind of information which you are passing to the QR code; it can be binary, numeric(only numbers) or alphanumeric (both numbers and alphabetical characters).
You can also specify the color of the QR dots and the background color by passing the following parameters.
url.png(qrstring, scale=6, module_color=[0, 0, 0, 128], background=[0xff, 0xff, 0xcc])